Print prime numbers between 1 to 100 in Java

In this blog, we are going to print the prime numbers between 1 to 100 using Java programming language. Here we are going to use conditional statements, loops, and basic operators to achieve the desired result.
Introduction?
A number that is more than one (1) and is divisible only by one (1) and the number itself is called a prime number. That is what we are going to figure out through code using Java.
Implementation⚒️
Initially start a codedamn playground in your preferred browser. A codedamn playground offers almost all the features required for a developer in the cloud. There is no need to set up the local environment. Also, it supports various languages which are shown below:
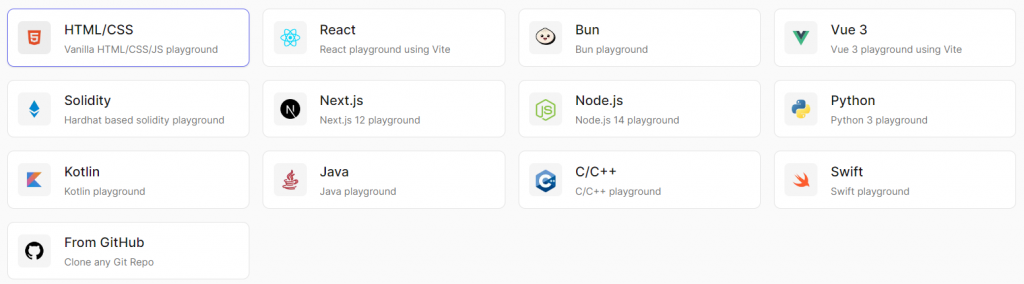
For this blog, we will stick to Java, hence let’s press Java and create a new playground. When you press Java you would be asked to provide a Playground Title. You are free to provide any name and hit the nice blue Create Playground button at the bottom.
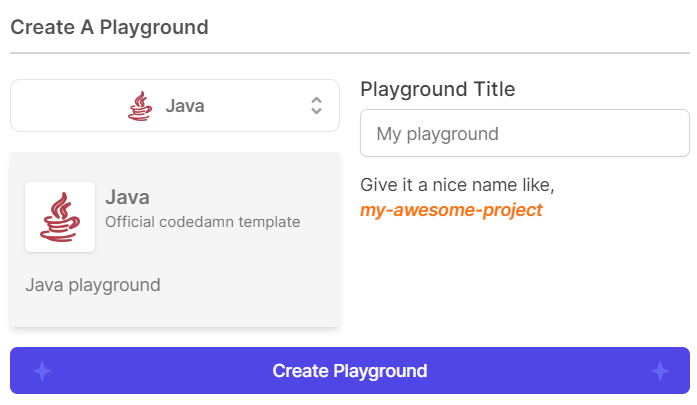
After you click on Create Playground, wait for a few seconds to load the editor in your browser. Ultimately you will land on a page similar to this:
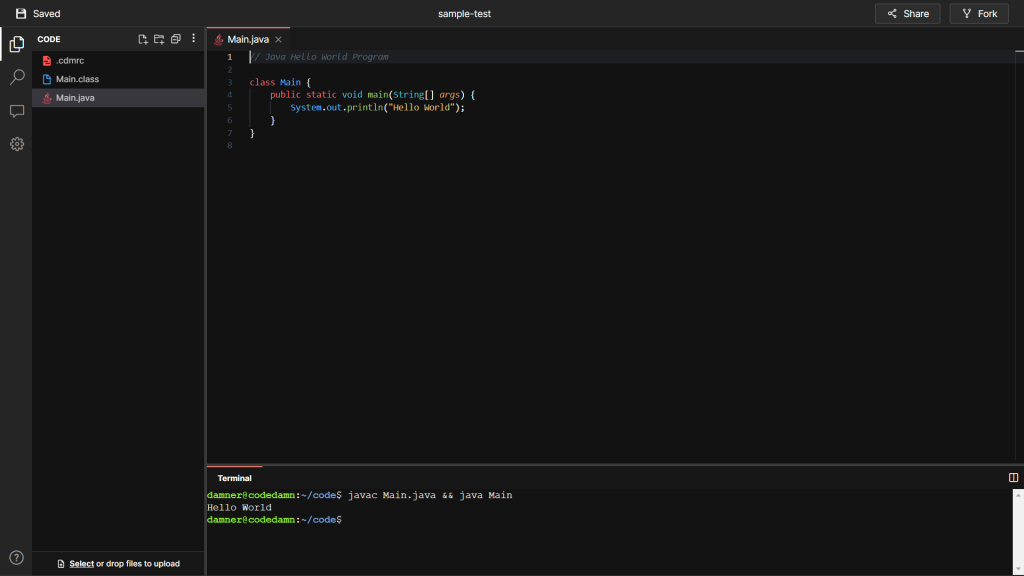
If you arrived at this stage without hiccups, then you are good further, else reiterate the steps slowly. to go to solve the problem of printing prime numbers between 1 to 100 in java.
Make sure that you are in the `Main.java` file and replace the below snippet with the existing content.
public class Main {
public static void main(String[] args) {
}
}
Code language: Java (java)
We know that Java is a class-based programming language, hence Every line of code that runs in Java must be inside a class. Also, a class name must start with a capital letter, in our case it is Main. Java is a case-sensitive language so be careful about it. But be careful that the name of the Java file must match the class name. This means adding a .java extension to the class name and saving the file.
Initialise variables?
Then let’s initialize the variables that we need to solve the problem. We need 4 variables namely, i, num, n, counter, and primeNumbers. The first three belong to the integer data type and the last one belongs to the string data type. The java implementation is as follows:
int i = 0; // Iterating variable (outer for-loop)
int num = 0; // Iterating Varible (inner for-loop)_
int n = 100; // Maximum limit (between 1 to 100)
int counter = 0; // Reinitialzed after every iteration
String primeNumbers = ""; // Store prime numbers
Code language: Java (java)
Comments in Java begin with //, so make sure to add comments wherever possible to make it easier for other developers to understand your code implementation.
Finding prime numbers?
Now we have to work on the logic of displaying the prime numbers. So for that initially we run an outer for loop to run over numbers between 1 to 100 (i). Then we set the counter variable (counter) to 0, after which run an inner for loop from the current number to 1, basically decrementing the variable (num). Then inside the inner for loop lies the conditional statement to check if the current number is divisible by itself and 1 (according to the definition).
Therefore, it is clear that the condition has to remain true only for 2 cases i.e. the value of the counter variable has to be 2 for every number between 1 to 100 to satisfy the condition of a prime number. Also whenever a number satisfies the condition of counter value equal to 2, then we add it as a string in the primeNumbers variable. The reason to use a string instead of an array is to reduce the time complexity. But let’s not discuss more regarding, time complexity as it is beyond the scope of the blog.
Now, this theoretical understanding has to be converted into code that is shown below:
for (i = 1; i <= n; i++) {
counter = 0;
for (num = i; num >= 1; num--) {
if (i % num == 0) {
counter = counter + 1;
}
}
if (counter == 2) {
//Append prime number as a string
primeNumbers = primeNumbers + i + " ";
}
}
Code language: Java (java)
Output?
Finally, we need to print the prime numbers for which we use the println function which is equivalent to printf(C)
, cout(C++)
, and print
(python). Here is the code for formatted output for better understanding:
System.out.println("Prime numbers from 1 to " + n +" are :");
System.out.println(primeNumbers);
Code language: Java (java)
Execution??♂️
To compile the code and run we use the terminal to execute the command:
javac Main.java && java Main
Code language: Bash (bash)
We get the output as shown below if there are no errors in code or logic. If there are any feel free to reiterate through the process slowly and you will be able to find the catch. Also never get demotivated if you face any error.

Conclusion?
We have successfully implemented printing the prime numbers between 1 to 100 using java. Here is the attached codedamn playground link for reference:
Also, this is not the only implementation to find prime numbers. There are numerous methods but I have used this method to keep it simple, clean, and understandable for all levels of coders. Feel free to use any other method that you feel better.
There is a comprehensive 11-hour Java course on codedamn. The best part about this course is that it is fully interactive. Tons of hands-on projects and exercises, lessons, articles, and quizzes to solidify your learnings.
Sharing is caring
Did you like what Sriniketh J wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: